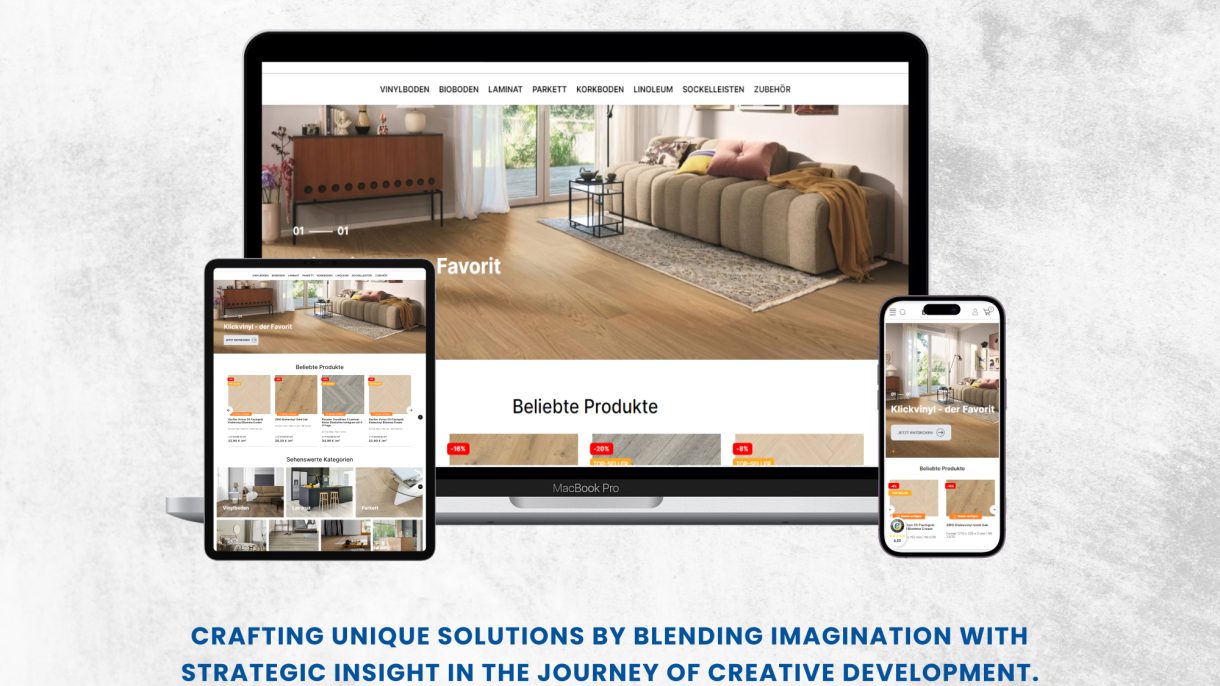
Frontend Development
- Client BODENIO
The task was to create a modern website that could be more interactive and have a functionality similar to Woocommerce, so React/NextJs was used with Woocommerce APIs for data management and other stuff.
Challenges
- Learning Curve:
- Requires understanding of both React and WooCommerce API functionalitiesFamiliarity with state management libraries (Redux, Context API) is beneficial.
- Security:
- Securely storing and handling API keys for WooCommerce.
- Implementing secure user authentication and payment processing.
- Data Management:
- Efficiently fetching and caching data from WooCommerce APIs to avoid overloading the server.
- Handling pagination and filtering for large datasets.
- State Management:
- Keeping track of user actions and shopping cart state across different components.
- Managing data updates and consistency across the React app.
- User Experience (UX):
- Creating a seamless shopping experience with smooth interactions and intuitive UI.
- Designing responsive layouts that adapt to different screen sizes.
Task
- Install React and necessary libraries (Axios for API calls, state management libraries like Redux or Context API).
- Configure a proxy server to avoid CORS issues (Cross-Origin Resource Sharing) if your React app is on a different domain than your WordPress site.
- Generate API keys for WooCommerce to access data securely.
- Fetching Data from WooCommerce APIs:
- Use libraries like Axios to make requests to WooCommerce REST API endpoints (products, categories, orders, etc.).
- Parse the JSON response and store the data in React state.
- Implement error handling for failed API requests.
- Building UI Components:
- Create React components to display product listings, product details, shopping cart, checkout flow, etc.
- Utilize React features like JSX, state management, and lifecycle methods for dynamic UI updates.
- Handling User Interactions:
- Implement user interactions like adding products to cart, updating quantities, managing user login/registration (potentially with JWT for secure authentication).
- Update the UI and state based on user actions.
- Integrating Payment Gateway:
- Choose a payment gateway compatible with WooCommerce and integrate it with your React app.
- Securely handle payment information and integrate it with the checkout process.
- Optimizing Performance:
- Implement techniques like code splitting, lazy loading, and caching to improve app loading speed.
- Consider using a library like React Query for efficient data fetching and caching.
Additional Tips
- Start with a simple project and gradually add features.
- Utilize existing libraries and resources for React and WooCommerce integration.
- Consider using a headless CMS approach where content management happens in WordPress and the frontend is built with React.
- Thoroughly test the functionality and user experience of your application.
These are some of the key tasks and challenges you might encounter when developing a React frontend with WooCommerce APIs. By understanding these points, you can approach your project with a clear plan and overcome potential hurdles.